1 什么是序列化和反序列化
当我们在写程序时,比如说我们自定义了一个实体类Person
,然后在程序中创建一个该实体类对象,并给对象赋了一些值,但是我们想将这些数据发给我们的其他的程序员朋友,让他们也可以调用我们创建的这个实体类并使用我们的数据,这个时候我们就需要使用到序列化和发序列化。
1.1 序列化
序列化就是将内存中运行的程序中的数据结构
数据存储到磁盘的文件
中,使其能够持久化存在电脑中,就算程序停止,这些数据也能一直存在。、
1.2 反序列化
顾名思义,也就是序列化的反操作,就是将已经存在磁盘文件中的数据读取到程序中继续使用的这么一个操作。
2 代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101
| #include <iostream> #include "string.h" #include <fstream>
using namespace std;
class Person { public: Person(char* name, int age) { strcpy(this->name, name); this->age = age; } Person() {} void showInfo() { cout << name << " " << age << endl; } int getAge() { return age; } char * getName() { return name; }
public: char name[10] { 0 }; int age = 0; };
void WriteBinaryFile(Person * ps,int size) { ofstream file("binary.txt",ios::out | ios::binary ); if (!file.is_open()) { cout << "文件打开失败" << endl; }
for (int i=0;i<size;i++) { file.write((char*)&ps[i],sizeof(Person)); }
file.close(); }
Person * ReadBinaryFile() { ifstream file("binary.txt", ios::in | ios::binary);
if (!file.is_open()) { cout << "文件打开失败" << endl; }
char* temp = new char[sizeof(Person)]; Person* ps = new Person[5]; int i=0; while (file.read(temp,sizeof(Person))) { Person p = *(Person*)temp; ps[i] = p; i++; }
file.close(); return ps; }
int main(int argc, char *argv[]) {
Person* ps2; ps2 = ReadBinaryFile(); for (int i=0; i<5; i++) { cout << ps2[i].getName() << " " << ps2[i].getAge() << endl; }
return 0; }
|
存储在文件中的内容的样子:

运行结果:
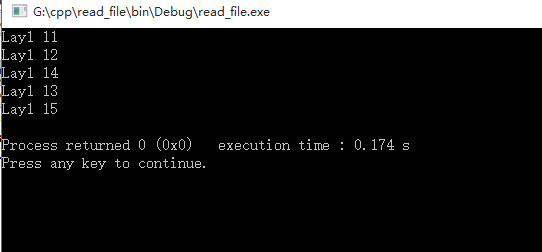
写在最后
欢迎大家关注鄙人的公众号【麦田里的守望者zhg】,让我们一起成长,谢谢。
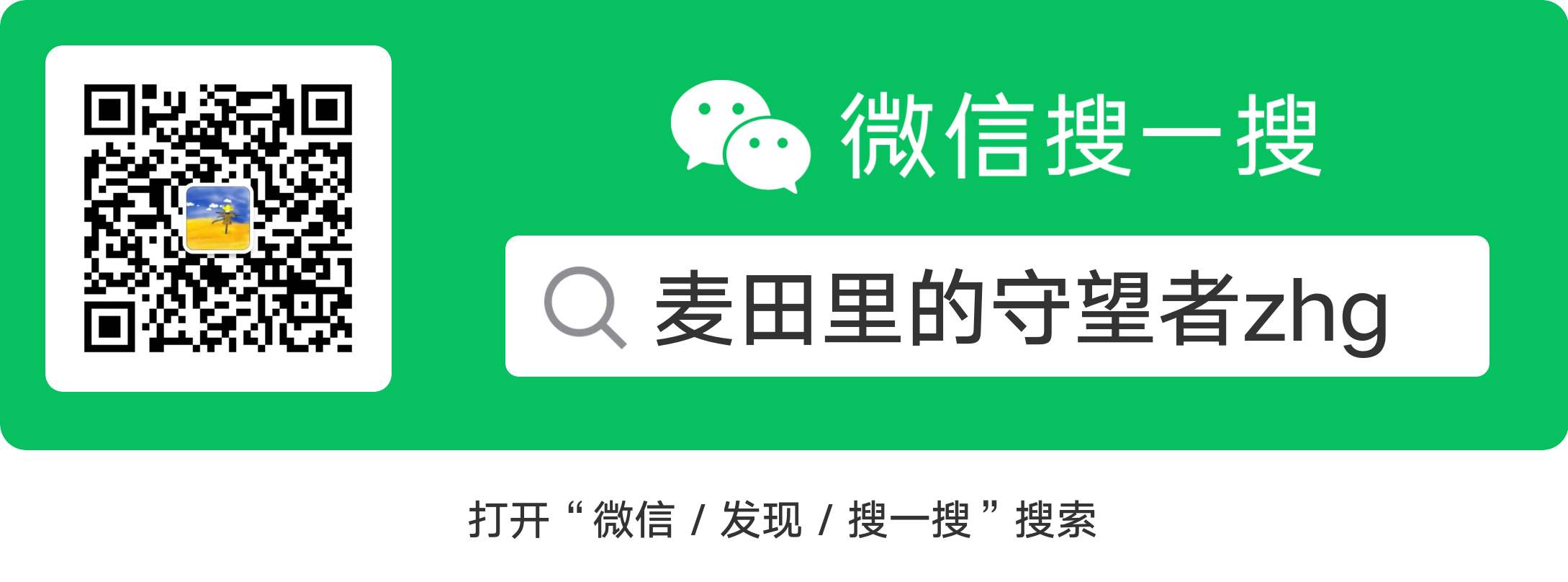